Proxy Pattern provides a surrogate or placeholder for another object in order to control access to it. You have an object that you want to get access to on which you want to invoke methods on and somehow interact with it but instead of being allowed to interact with that object you have a proxy which interacts with that object. With proxy pattern we are trying to solve specific problems that all are access related, so you put a proxy in front of something that you want to allow people to access but you have the proxy so you can control access to that thing.
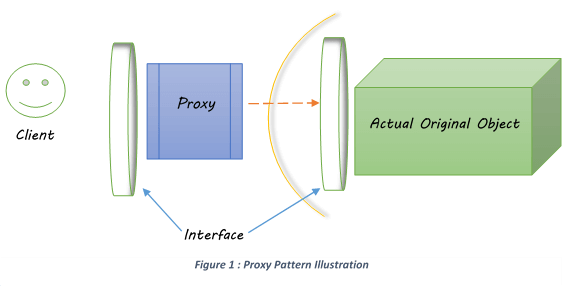
Proxies are mainly categorized into remote, virtual and protection proxy. A remote proxy is suggested to be used when you want to access a resource which is remote. A virtual proxy controls access to a resource that is expensive to create like caching. Consider an object which is expensive to create, when you put a proxy before the object it controls creation of the object only when its necessary. A protection proxy is like an access management, consider a resource which is limited to user roles when you put a proxy before that, it would make sure only the users who have required roles have access to underlying resource.
Proxy pattern adds additional behavior with an intent to control the access of underlying object. Proxy pattern doesn’t change the interface you want to interact with, it behaves like it was the actual resource by adding additional behavior just before calling to the resource.
Example
For example, imagine the text in a book is represented by a string which has the entire information and is huge. We have a class BookParser which does the operations on this string and it is passed in constructor for the class so that we can do something like bp.NumberOfPages(), bp.NumberOfChapters(). The values of these methods are precomputed when the BookParser is created from the constructor, so these operations are cheap. Let say we have different places in our code which instantiates the BookParser even though we don’t call these methods. This becomes a performance bottle neck as we are instantiating BookParser several places in our application which is an expensive operation, but we are not actually using its methods.
This can be solved by sticking a proxy before whoever calls the BookParser which controls the creation of it. Instead of client directly interacting with the BookParser client interacts with proxy which interacts with BookParser but will not instantiate it until client access the methods of the BookParser. It looks something like below.
Code
Below is the implementation of above code in C#
The proxy has exactly the same interface of the book parser so the client can keep behaving the proxy as a book parser. Once one of the methods of the book parser are invoked the proxy creates a book parser and returns the value and maintains reference of it so that for future calls to book parser it doesn’t take the same time as the first call.
UML Diagram
We have an interface ISubject having a method request implemented by a concrete class RealSubject which provides the implementation of the method request which in our example was a BookParser class with NumberOfPages method. There is proxy concrete class which follows the same interface of RealSubject and also Proxy has a RealSubject which means that it can delegate to RealSubject and also responsible for controlling the access to RealSubject.
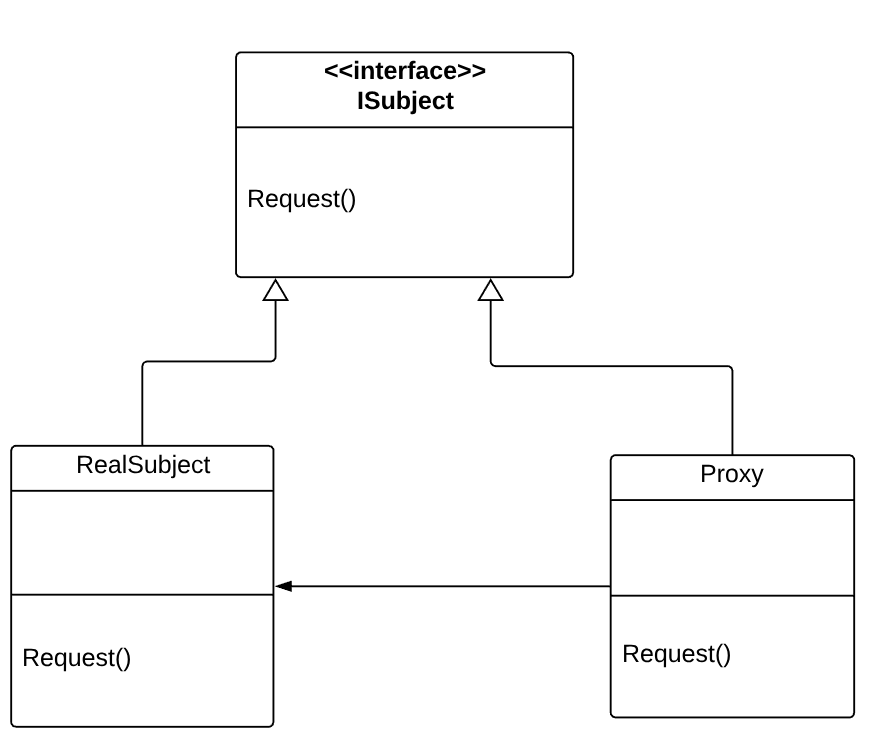
Proxy controls the access to RealSubject and it follows the same interface. Proxy doesn’t necessarily have to pass the instantiation of the Real Subject.
GitHub Link : ProxyPattern
#9DayOf100DaysOfCode
Comments