Command Pattern
The Command pattern encapsulates the request as an object thereby letting you parameterize other objects with different requests queue or log request and support undoable operations. The key thing to understand here is its not the sender who is sending the request nor the receiver who is consuming it is encapsulated, its the request itself is being encapsulated. Command pattern also states that if I have a command which does something it should also be able to undo the command.
Example
Consider example of Philips smart lighting which are Wifi connected light bulbs. We have a switch controller for the bulb which essentially let the user to On, dim up, dim down and turn off the light. We can use the Philip hue app on the mobile where we can program the different buttons on the controller to be connected to different lights. Here the invoker is the controller and the receiver are the light bulb.
The command for each of the buttons in the invoker are configurable by the user and not hard-coded which means by pressing off button on the controller all the bulbs in the home can be turned off.
An Example for the undo operation would be something like a photoshop application where every action user performs is stored as a command and every command has a undo operation on it, so when user calls undo the most recent command from the list is taken and undo operation is invoked on it.
UML Diagram
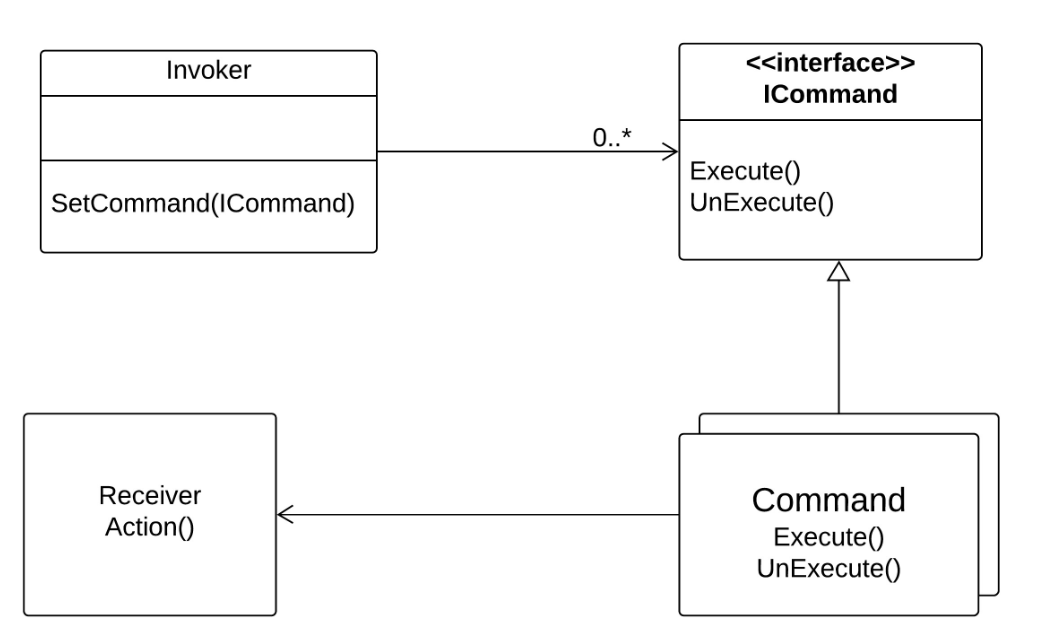
Invoker has a reference to zero to many ICommand. In our example Invoker is a controller and it has commands. Each concrete command class has a receiver on which they act on. Here the receiver is a light bulb. We have SetCommand(ICommand) method on Invoker class. The ICommand has definitions for execute () and unexecute () where execute will execute the command and unexecute will support the undo operation of the same. Concrete command classes will provide the implementations for these methods. The methods on the receiver are completely dependent on the scenario. Here we have action method which essentially performs the action on the light bulb.
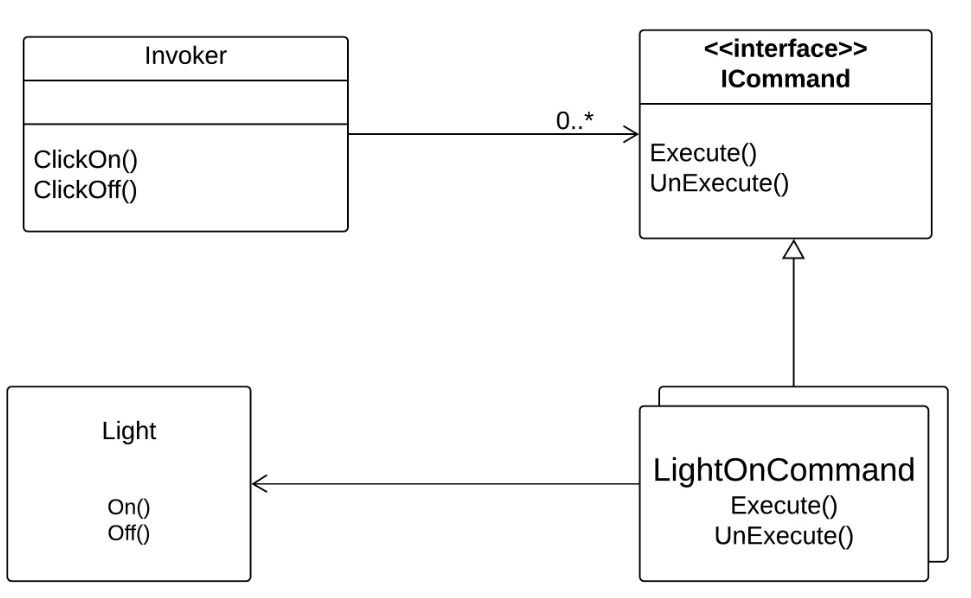
Code
We have an Invoker class which has methods for ClickOn and ClickOff methods and it has a reference to ICommand interface. In our example we have LightOn and LigthOff command classes inheriting from ICommand. These two concrete classes provide implementations for execute and unexecute methods of the interface. The action of turning on the light is encapsulated in these classes which lets us parametrize other requests.
GitHub: CommandPattern
#18DayOf100DaysOfCode
Comments